일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | ||||||
2 | 3 | 4 | 5 | 6 | 7 | 8 |
9 | 10 | 11 | 12 | 13 | 14 | 15 |
16 | 17 | 18 | 19 | 20 | 21 | 22 |
23 | 24 | 25 | 26 | 27 | 28 |
- 내일배움투어
- 국비지원파이썬
- ios
- 부트캠프
- Udemy
- 고용노동부국비지원
- 개발
- IT개발캠프
- 앱개발
- 인사이드아웃
- 러닝핏인강
- 0원코딩인강
- K디지털크레딧
- 내일배움카드인강
- 스나이퍼팩토리
- 웹개발
- 러닝핏습관챌린지
- 플러터
- 유데미
- 웅진씽크빅
- 국비코딩
- 내일배움카드사용처
- K디지털기초역량훈련
- 코딩국비지원
- Flutter
- 안드로이드
- 러닝핏
- 습관챌린지
- 개발자부트캠프
- 국비지원코딩
- Today
- Total
매일 땡기는 마라 코딩
[9주 완성 프로젝트 캠프 : 플러터(유데미x스나이퍼팩토리)] 2일차 과제 본문
요구사항
- 기분을 나타내는 위젯을 3가지 이상 만들고, 페이징이 가능하게 해야 한다. (gradient, radius 필수)
- ListTile 위젯을 사용하지 않고, 동일한 결과물을 만들어야 한다. (위아래 구분하는 구분선은 Divider로)
내가 해야 하는 건?
- gradient, Radius, Divider에 대해 자세히 찾아 본다.
- margin, padding 등의 속성값에 대해 알아 본다.
사전 지식
"EdgeInsets"
paddig, margin의 값을 조정할 때 사용한다. all, only 등 여러 옵션이 있다.
all: 전체 영역 여백 지정
margin: EdgeInsets.all(8)
only: 특정 영역 여백 지정
margin: EdgeInsets.only(top: 230)
fromLTRB: (왼, 위, 오, 아) 순서로 원하는 값의 여백 지정
padding: EdgeInsets.fromLTRB(0, 10, 5, 17)
symmetric: 수평(horizontal), 수직(vertical) 기준 여백 지정
padding: EdgeInsets.symmetric(vertical: 100)
"Expanded"
위젯을 부모 요소 영역의 최대 사이즈까지 확장해 준다.
Row 안에 있는 위젯은 가로로, Column 안에 있는 위젯은 세로로 확장된다.
child가 부모보다 작던 크던 상관없이 최대 사이즈로 확장한다.
flex 속성 값을 지정해 확장 비율을 설정할 수 있다.
Row( children: [ Expanded ( flex: 2, child: Container( color: Colors.red ), ), ], )
"alignment"
alignment는 Container와 같은 위젯의 자식 위젯(child)을 원하는 위치에 배치하기 위해 사용한다.
x,y의 좌표값, Center와 같은 명령어로 위치 지정이 가능하다.
자식 위젯이 다수(children)면 사용 불가능하며, Row, Column 안에 있는 Container는 children이 아니어도 사용 불가능.
alignment: Alignment.center
alignment: Alignment.(0.1, 3.3)
그 외
- width: double.infinity - 가로 폭을 부모 요소에 꽉 차게 만든다. 무한대로 확장.
- radius: 40 - 반지름을 지정한다.
코드
main.dart
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
body: SafeArea(
child: Center(
child: Column(
children: [
Container(
width: double.infinity,
height: 80,
margin: EdgeInsets.only(top: 230),
child: Column(
children: [
Container(
child: Text(
'오늘의 하루는',
style: TextStyle(
fontSize: 32,
fontWeight: FontWeight.bold,
),
),
),
Container(
child: Text(
'어땠나요?',
style: TextStyle(
fontSize: 20,
),
),
),
],
),
),
Container(
width: 300,
height: 200,
child: PageView(
children: [
Container(
margin: EdgeInsets.all(8),
alignment: Alignment.center,
child: Text(
'우울함',
style: TextStyle(
color: Colors.white,
fontSize: 20,
),
),
decoration: BoxDecoration(
borderRadius: BorderRadius.circular(8),
gradient: LinearGradient(
begin: Alignment.centerLeft,
end: Alignment.centerRight,
colors: [
Colors.black,
Colors.white,
],
),
),
),
Container(
alignment: Alignment.center,
child: Text(
'행복함',
style: TextStyle(
color: Colors.white,
fontSize: 20,
),
),
decoration: BoxDecoration(
borderRadius: BorderRadius.circular(8),
gradient: LinearGradient(
begin: Alignment.centerLeft,
end: Alignment.centerRight,
colors: [
Colors.orange,
Colors.yellow,
]),
),
),
Container(
alignment: Alignment.center,
child: Text(
'상쾌함',
style: TextStyle(
color: Colors.white,
fontSize: 20,
),
),
decoration: BoxDecoration(
borderRadius: BorderRadius.circular(8),
gradient: LinearGradient(
begin: Alignment.centerLeft,
end: Alignment.centerRight,
colors: [
Colors.blue,
Colors.green,
],
),
),
),
],
),
),
Divider(),
Container(
width: double.infinity,
height: 110,
margin: EdgeInsets.only(top: 8),
padding: EdgeInsets.all(8),
decoration: BoxDecoration(
color: Colors.blue,
),
child: Row(
children: [
Expanded(
flex: 2,
child: CircleAvatar(
radius: 40,
backgroundImage:
NetworkImage("https://picsum.photos/100/100"),
),
),
Expanded(
flex: 4,
child: Container(
margin: EdgeInsets.fromLTRB(5, 12, 0, 12),
alignment: Alignment.centerLeft,
child: Column(
children: [
Text(
'라이언',
style: TextStyle(
color: Colors.white,
fontSize: 21,
),
),
Text(
'개임개발',
style: TextStyle(
color: Colors.white,
),
),
Text(
'C#, Unity',
style: TextStyle(
color: Colors.white,
),
),
],
),
),
),
Expanded(
flex: 1,
child: Icon(
Icons.add,
color: Colors.white,
),
),
],
),
)
],
),
),
),
),
);
}
}
결과
회고(코드리뷰)
난 이렇게 시간이 많이 걸릴지 몰랐지,,,
생소한 언어로 코딩을 하다 보니 감이 더 안 온다.
Container로 위젯을 감쌀 생각을 못 해서 엄청나게 헤매버렸음...
그래도 하루하루 지식이 쌓여가는 기분이다. 뿌듯.
과제 제출 다음 날, 강의를 듣고 있는데 담당 멘토님인 신디 멘토님이 말을 거셨다.
과제 코드리뷰 후에 간단하게 수정 사항을 말씀해 주셨는데,
틀린 줄 알고 사실 조금 쫄았었다 ㅎㅎ...
Expanded를 사용한 부분을 Spacer로 대체해 보는 것이 어떻냐고 하시면서 잘 정리된 블로그를 알려 주고 가셨다.
Flutter : Spacer 이용하기
Spacer 이용하기 Flutter에서 Spacer는 유연한 빈 공간을 만들기 위해 사용되는 위젯입니다. 이 위젯을 Row나 Column과 같은 Flex container 안에 배치하면, 이전 위젯과 다음 위젯 사이에 자동으로 공간이 생
swjs.tistory.com
Spacer는 자식 위젯들을 한 줄로 나열하는 Row, Column 내에서 사용한다.
Spacer를 사용하면 하나씩 flex를 지정할 필요 없이, 빈 공간에 flex를 주어 유연하게 원하는 위치에 배치할 수 있는 듯하다.
Expanded는 위젯의 크기를, 지정한 비율에 맞게 확장하여 간격을 조정한다.
Spacer는 위젯과 위젯 사이, 빈 공간을 지정한 비율에 맞게 확장하여 간격을 조정한다.
공통점은 부모 위젯의 범위를 꽉 채우도록 확장한다는 것과, Expanded, Spacer가 설정되어 있지 않은 위젯이 우선적으로 크기를 가져간다는 것?
열심히 찾아보긴 했는데 정답인지는 모르겠다 ㅎ...
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
body: SafeArea(
child: Center(
child: Column(
children: [
Container(
width: double.infinity,
height: 80,
margin: EdgeInsets.only(top: 230),
child: Column(
children: [
Container(
child: Text(
'오늘의 하루는',
style: TextStyle(
fontSize: 32,
fontWeight: FontWeight.bold,
),
),
),
Container(
child: Text(
'어땠나요?',
style: TextStyle(
fontSize: 20,
),
),
),
],
),
),
Container(
width: 300,
height: 200,
child: PageView(
children: [
Container(
margin: EdgeInsets.all(8),
alignment: Alignment.center,
child: Text(
'우울함',
style: TextStyle(
color: Colors.white,
fontSize: 20,
),
),
decoration: BoxDecoration(
borderRadius: BorderRadius.circular(8),
gradient: LinearGradient(
begin: Alignment.centerLeft,
end: Alignment.centerRight,
colors: [
Colors.black,
Colors.white,
],
),
),
),
Container(
alignment: Alignment.center,
child: Text(
'행복함',
style: TextStyle(
color: Colors.white,
fontSize: 20,
),
),
decoration: BoxDecoration(
borderRadius: BorderRadius.circular(8),
gradient: LinearGradient(
begin: Alignment.centerLeft,
end: Alignment.centerRight,
colors: [
Colors.orange,
Colors.yellow,
]),
),
),
Container(
alignment: Alignment.center,
child: Text(
'상쾌함',
style: TextStyle(
color: Colors.white,
fontSize: 20,
),
),
decoration: BoxDecoration(
borderRadius: BorderRadius.circular(8),
gradient: LinearGradient(
begin: Alignment.centerLeft,
end: Alignment.centerRight,
colors: [
Colors.blue,
Colors.green,
],
),
),
),
],
),
),
Divider(),
Container(
width: double.infinity,
height: 100,
margin: EdgeInsets.only(top: 8),
padding: EdgeInsets.all(15),
decoration: BoxDecoration(
color: Colors.blue,
),
child: Row(
children: [
CircleAvatar(
radius: 40,
backgroundImage:
NetworkImage("https://picsum.photos/100/100"),
),
Container(
margin: EdgeInsets.only(left: 8),
child: Column(
children: [
Text(
'라이언',
style: TextStyle(
color: Colors.white,
fontSize: 21,
),
),
Text(
'개임개발',
style: TextStyle(
color: Colors.white,
),
),
Text(
'C#, Unity',
style: TextStyle(
color: Colors.white,
),
),
],
),
),
Spacer(flex: 2),
Icon(
Icons.add,
color: Colors.white,
),
],
),
)
],
),
),
),
),
);
}
}
Spacer를 사용하니 훨씬 간결한 코드로 결과물을 완성할 수 있었다.
코드를 제대로 작성한 게 맞는지 잘 모르겠어서 고치느라 시간을 많이 잡아먹었었는데,
멘토님이 과제 확인 후에 어느 부분을 수정하면 좋을지 짚어 주셔서 굉장히 나이스였다.
천재만재 신디 멘토님 감삼다.
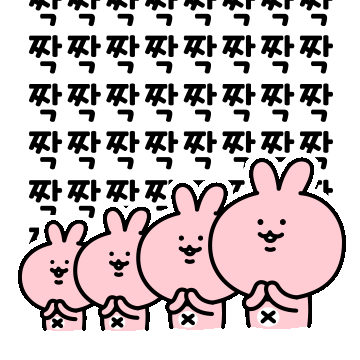
참고 자료
- https://blog.naver.com/wofkd14/222985925142
- https://nx006.tistory.com/8
- https://codingapple.com/unit/flutter-margin-padding-sizedbox/
- https://padro.tistory.com/162
- https://learncom1234.tistory.com/44
- https://negabaro.github.io/archive/flutter-painting-EdgeInsets
본 후기는 유데미-스나이퍼팩토리 9주 완성 프로젝트캠프 학습 일지 후기로 작성 되었습니다.
#유데미 #udemy #스나이퍼팩토리 #웅진씽크빅 #인사이드아웃 #IT개발캠프 #개발자부트캠프 #웹개발 #앱개발 #플러터 #flutter #개발 #안드로이드 #ios #단기캠프
'Flutter' 카테고리의 다른 글
[9주 완성 프로젝트 캠프 : 플러터(유데미x스나이퍼팩토리)] 3일차 과제 - 유튜브 뮤직 클론코딩 (5) | 2023.10.22 |
---|---|
[9주 완성 프로젝트 캠프 : 플러터(유데미x스나이퍼팩토리)] 3일차 과제 - 스타벅스 클론코딩 (0) | 2023.09.21 |
[9주 완성 프로젝트 캠프 : 플러터(유데미x스나이퍼팩토리)] 1일차 과제 (0) | 2023.09.20 |
[9주 완성 프로젝트 캠프 : 플러터(유데미x스나이퍼팩토리)] #0-2. Git (0) | 2023.09.19 |
[9주 완성 프로젝트 캠프 : 플러터(유데미x스나이퍼팩토리)] #0-1. 사전 준비 (0) | 2023.09.18 |